UploadFile#
For the UploadFile method, we recommend to use the media.green-api.com host
The method is designed to upload a file to the cloud storage, which can be sent using the sendFileByUrl method. It also allows you to avoid errors in receiving files from external storages and speed up sending messages, for example, in a mailing script.
An alternative way to send a file by uploading from disk: sendFileByUpload.
Link lifetime is 15 days.
The maximum file size that can be sent is 100 MB.
The type of file you send and how you send it is determined by the file extension.
Using the UploadFile Method
It is recommended to use the method when sending messages of the same type:
- Using the UploadFile method, upload the required file to the Green-API storage, receive a link to the file in the response.
- Use the SendFileByUrl method to send using the received link.
How to obtain information about the file upload deadline
To get information about the file upload deadline, you need to retrieve information from the link header
To do this:
- Obtain the link to the file
-
Execute a
HEAD
request with the obtained link -
From the result, retrieve the value of the
X-Amz-Expiration
field - The obtained value indicates until when the link will be available.
For example, if you receive the value of the X-Amz-Expiration
field, which is expiry-date="Thu, 25 Apr 2024 00:00:00 GMT"
. This denotes that the link is available until 04/25/2024 00:00:00 in the zero time zone.
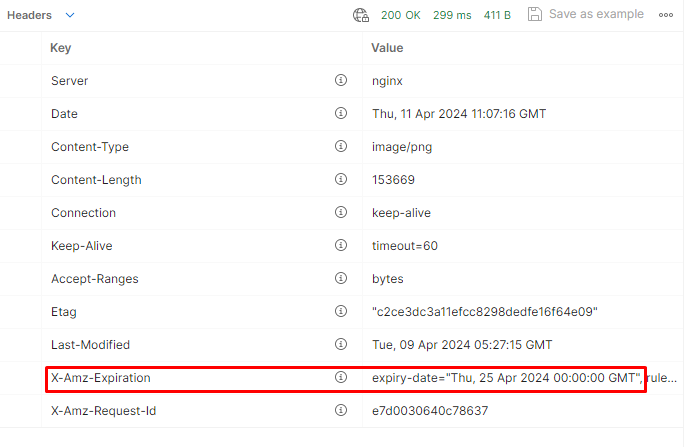
Request#
To download a file, you need to make a request to the address:
POST {{MediaUrl}}/waInstance{{idInstance}}/uploadFile/{{apiTokenInstance}}
See the Before you start section to obtain the MediaUrl
, idInstance
and apiTokenInstance
query parameters.
Request parameters#
You must specify the file itself as the body of the request.
- In the request header, you must specify the file type
Content-Type
in accordance with the List of MIME types. - If the file is not in the list of MIME types, you can pass the GA-Filename header, specifying the file name with the extension. This will allow you to upload files such as .go or .py.
- If the system cannot determine the file type, it will be sent with the default type (binary file): .bin
Parameter | Type | Mandatory | Description |
---|---|---|---|
file | binary | Yes | Upload file |
Request body example#
Code examples
import requests
url = "{{MediaUrl}}/waInstance{{idInstance}}/uploadFile/{{apiTokenInstance}}"
payload = "<file contents here>"
headers = {
'Content-Type': 'image/jpeg'
}
response = requests.request("POST", url, headers = headers, data = payload)
print(response.text.encode('utf8'))
curl --location '{{MediaUrl}}/{{idInstance}}/uploadFile/{{apiTokenInstance}}' \
--header 'Content-Type: image/jpeg' \
--data-binary "@/Users/user/Desktop/fileExample.jpeg"
var file = new File("/Users/user/Desktop/fileExample.jpeg");
var restTemplate = new RestTemplate();
var requestUrl = new StringBuilder();
requestUrl
.append("https://media.greenapi.com")
.append("/waInstance").append({{idInstance}})
.append("/uploadFile/")
.append({{apiTokenInstance}});
var byteArrayResource = new ByteArrayResource(Files.readAllBytes(file.toPath()));
var headers = new HttpHeaders();
headers.setContentType(MediaTypeFactory.getMediaType(file.getName())
.orElse(MediaType.APPLICATION_OCTET_STREAM));
var requestEntity = new HttpEntity<>(byteArrayResource, headers);
var response = restTemplate.exchange(requestUrl.toString(), HttpMethod.POST, requestEntity, String.class);
System.out.println(response);
var file = new File("/Users/user/Desktop/fileExample.jpeg");
var requestUrl = new StringBuilder();
requestUrl
.append("https://media.greenapi.com")
.append("/waInstance").append({{idInstance}})
.append("/uploadFile/")
.append({{apiTokenInstance}});
var response = Unirest.post(requestUrl.toString())
.header("Content-Type", Files.probeContentType(file.toPath()))
.body(Files.readAllBytes(file.toPath()))
.asString();
System.out.println(response);
<?php
// Need to use SDK https://github.com/green-api/whatsapp-api-client-php
require './vendor/autoload.php';
use GreenApi\RestApi\GreenApiClient;
define( "ID_INSTANCE", "1101123456" );
define( "API_TOKEN_INSTANCE", "d75b3a66374942c5b3c019c698abc2067e151558acbd412345" );
$greenApi = new GreenApiClient( ID_INSTANCE, API_TOKEN_INSTANCE );
$result = $greenApi->sending->uploadFile(
'C:\Games\PicFromDisk.png'
);
print_r( $result->data );
Response#
Response fields#
Field | Type | Description |
---|---|---|
urlFile | string | Link to uploaded file |
Response body example#
{
"urlFile": "https://sw-media-out.storage.yandexcloud.net/1101123456/c1aabd48-c1c2-49b1-8f2d-f575a41777be.jpg"
}
Code example using methods uploadFile + sendFileByUrl#
<?php
// Need to use SDK https://github.com/green-api/whatsapp-api-client-php
require './vendor/autoload.php';
use GreenApi\RestApi\GreenApiClient;
define( "ID_INSTANCE", "1101123456" );
define( "API_TOKEN_INSTANCE", "d75b3a66374942c5b3c019c698abc2067e151558acbd412345" );
$greenApi = new GreenApiClient( ID_INSTANCE, API_TOKEN_INSTANCE, 'http://127.0.0.1:8080' );
$result = $greenApi->sending->uploadFile(
'C:\Games\PicFromDisk.png'
);
print_r( $result->data );
$result = $greenApi->sending->sendFileByUrl(
'11001234567@c.us',
$result->data->urlFile,
'googlelogo_color_272x92dp.png',
'Google logo'
);
print_r( $result->data );
Errors UploadFile#
For a list of common errors for all methods, see the Common errors section
HTTP code | Error ID | Description |
---|---|---|
400 | file should not be empty | The user submitted an empty file. The uploaded file must not be empty. |
413 | request entity too large | Occurs when sending files in 1C processing (version 8.3.22.1923). Possible solution: Change 1C version. |